Referencing LaTeX objects from Matplotlib
Recently, I wanted to reference an equation in a LaTeX document from a figure created using matplotlib. Luckily, matplotlib has a PGF backend that makes this possible. Here’s an example:
main.tex
:
\documentclass[12pt]{article}
\usepackage{amsmath}
\usepackage{pgf}
\begin{document}
\begin{equation}
\label{eq:foo}
e^{i\pi} + 1 = 0
\end{equation}
\begin{figure}
\centering
\resizebox{3in}{3in}{
\input{figure.pgf}
}
\caption{This is the caption.}
\end{figure}
\end{document}
So we have an labeled equation, and we’d like to create the file figure.pgf
so that the equation can be referenced in the figure.
make_figure.py
:
import numpy as np
import matplotlib as mpl
mpl.use('pgf')
pgf_with_custom_preamble = {
"text.usetex": True, # use inline math for ticks
"pgf.rcfonts": False, # don't setup fonts from rc parameters
"pgf.preamble": [
"\\usepackage{amsmath}", # load additional packages
]
}
mpl.rcParams.update(pgf_with_custom_preamble)
import matplotlib.pyplot as plt
plt.figure(figsize=(4,4))
plt.plot(np.random.randn(10), label="Equation \\eqref{eq:foo}")
plt.grid(ls=":")
plt.legend(loc=2)
plt.savefig("figure.pgf", bbox_inches="tight")
I’ve borrowed some of the settings from the matplotlib pgf page. You can apparently get quite deep into the pgf settings in matplotlib (see here for example).
Now, finally run,
python make_figure.py
pdflatex main
pdflatex main
That not a typo — you must run pdflatex
twice to get the references correct. Here’s the output:
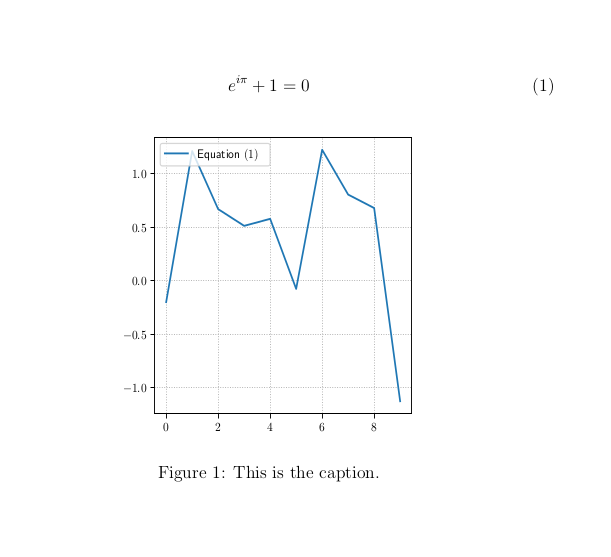
Further, if you add \usepackage{hyperref}
to main.tex
, the equation reference in the figure is hyperlinked to the equation itself!